C# 循环语句(for, while, do-while, foreach)
|
admin
2024年12月11日 19:12
本文热度 1016
|
循环语句是编程中用于重复执行一段代码直到满足特定条件的控制结构。在 C# 中,循环语句包括 for
、while
、do-while
和 foreach
。本课程将逐一介绍这些循环语句的特点和使用场景,并通过示例加深理解。
1. for 循环
应用特点
应用场景
示例
// 简单的计数循环
for (int i = 0; i < 10; i++) {
Console.WriteLine("计数值:" + i);
}
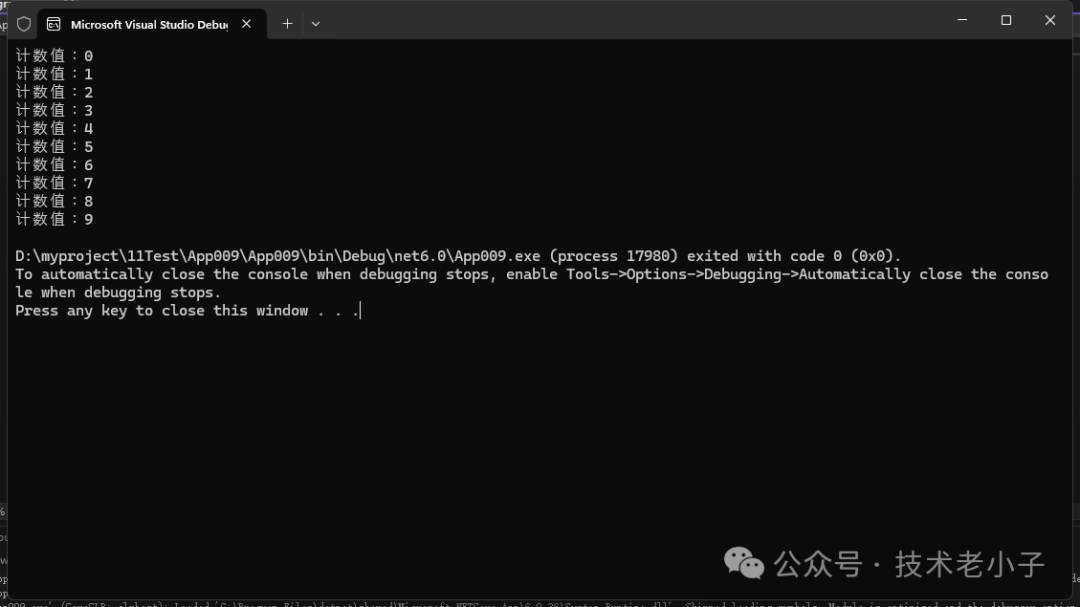
// 遍历数组
int[] array = { 1, 2, 3, 4, 5 };
for (int i = 0; i < array.Length; i++) {
Console.WriteLine("数组元素:" + array[i]);
}
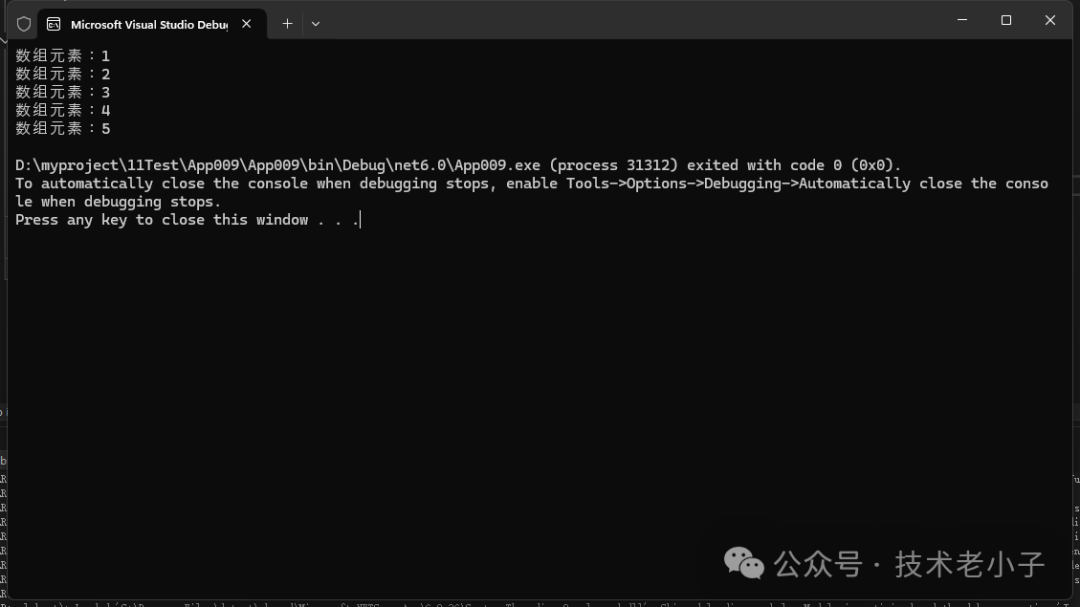
2. while 循环
应用特点
while
循环在每次迭代开始前检查条件。
适用于循环次数未知的情况。
应用场景
等待用户输入或外部事件触发。
持续检查某个条件是否满足。
示例
// 条件控制循环
int i = 0;
while (i < 10) {
Console.WriteLine("计数值:" + i);
i++;
}
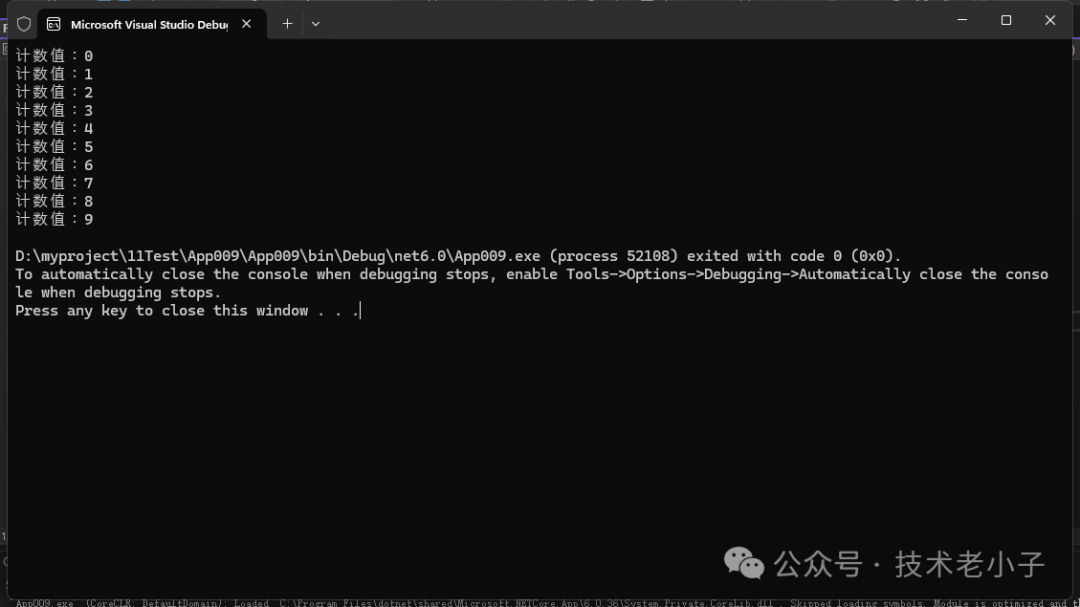
// 用户输入控制循环
string userInput;
do {
Console.WriteLine("请输入 'exit' 退出循环:");
userInput = Console.ReadLine();
} while (userInput != "exit");
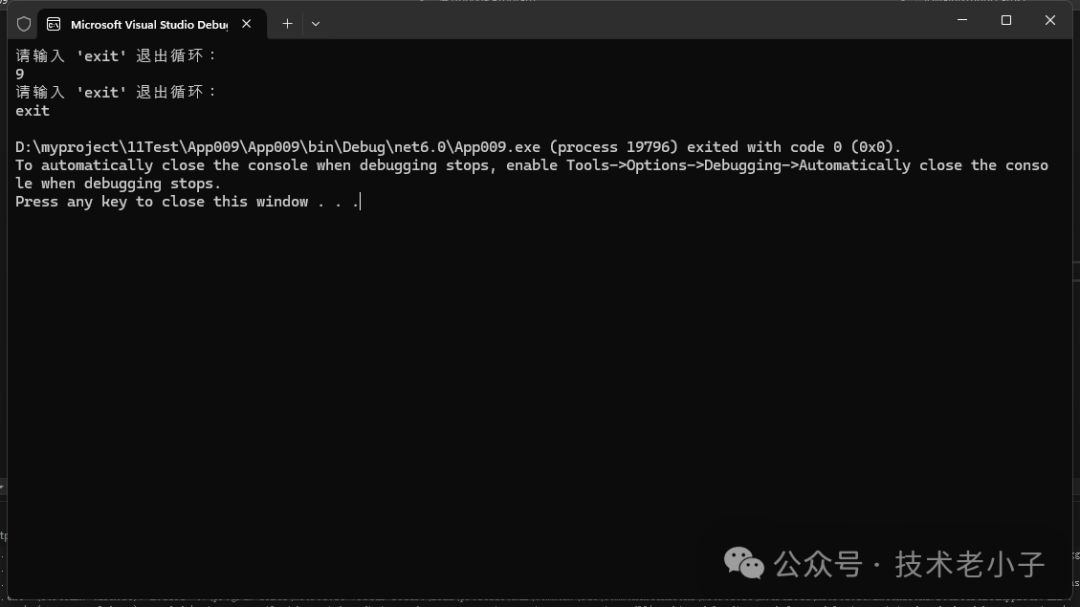
3. do-while 循环
应用特点
应用场景
示例
// 至少执行一次的循环
int count = 0;
do {
count++;
Console.WriteLine("执行次数:" + count);
} while (count < 5);
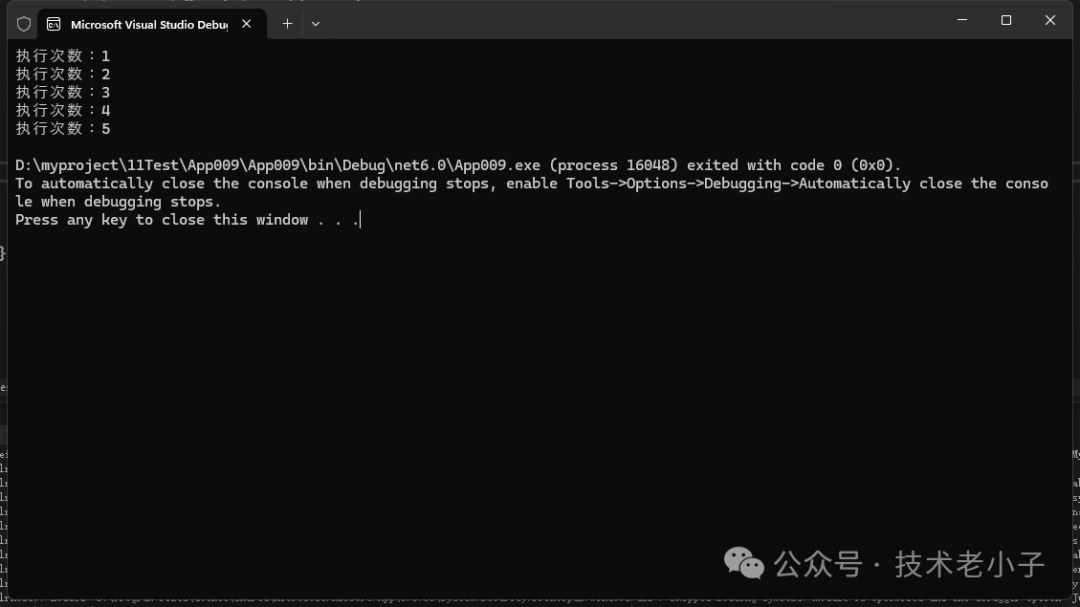
4. foreach 循环
应用特点
foreach
循环用于简化集合或数组的遍历。
直接操作集合中的每个元素,无需使用索引。
应用场景
读取集合中的所有元素。
不需要修改集合中元素的情况。
示例
// 遍历集合
List<string> names = new List<string> { "Alice", "Bob", "Charlie" };
foreach (string name in names) {
Console.WriteLine("姓名:" + name);
}
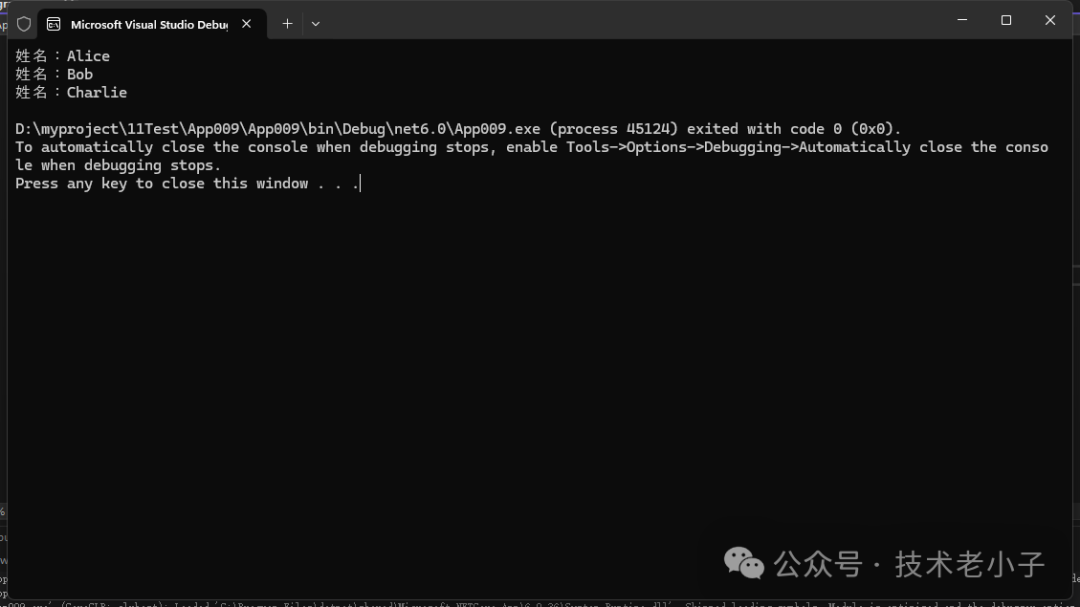
// 遍历字典
Dictionary<string, string> capitals = new Dictionary<string, string> {
{ "France", "Paris" },
{ "Germany", "Berlin" }
};
foreach (KeyValuePair<string, string> item in capitals) {
Console.WriteLine("国家:" + item.Key + ", 首都:" + item.Value);
}
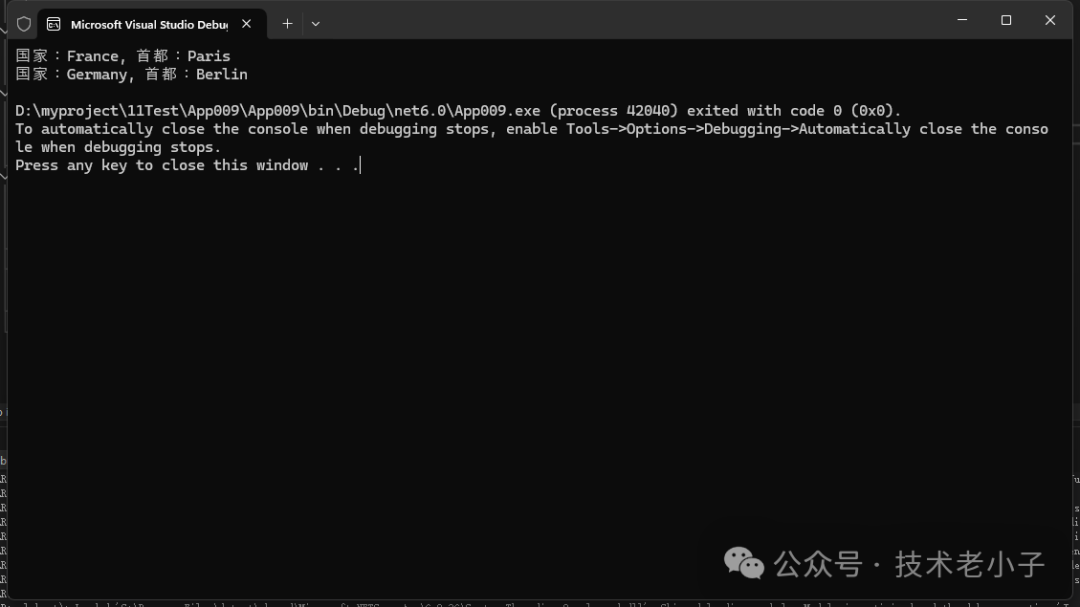
结语
C# 中的循环语句是编写高效、可读性强的代码的基础。选择合适的循环结构可以简化代码逻辑,提高程序性能。通过本课程的学习,您应该能够灵活运用不同的循环语句来处理各种重复执行的任务。
该文章在 2024/12/12 10:22:48 编辑过